Python performance analysis with Profile module
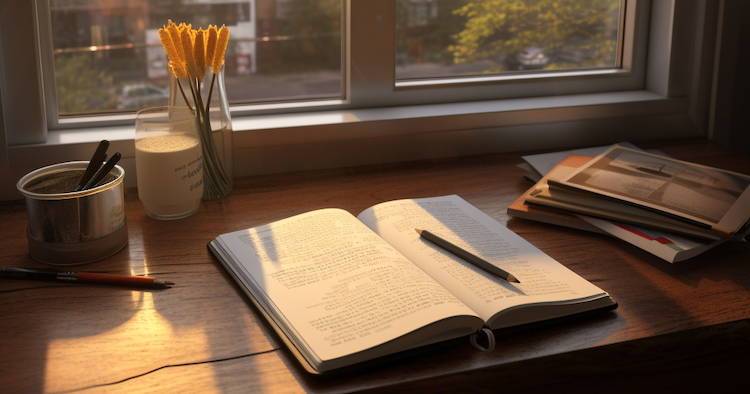
#!/usr/bin/env python
from random import randint
from cProfile import Profile
from pstats import Stats
def insert_value(array, value):
for i, existing in enumerate(array):
if existing > value:
array.insert(i, value)
return
array.append(value)
def insertion_sort(data):
result = []
for value in data:
insert_value(result, value)
return result
if __name__ == '__main__':
max_size = 10 ** 4
data = [randint(0, max_size) for _ in range(max_size)]
test = lambda: insertion_sort(data)
profiler = Profile()
profiler.runcall(test)
stats = Stats(profiler)
stats.strip_dirs()
stats.sort_stats('cumulative')
stats.print_stats()
Leave a message